Hello. My name is Ben Smith and I head up Product Management for Experience, Software, and Developer Programs at Sonos.
I wanted to get more feedback from you all on your expectations for Shuffling tracks in your queue. I have reviewed the feedback on ask.sonos.com and received e-mails from a few of you (via our CEO John MacFarlane), but I wanted to get a better sense from those of you who use Shuffle, how your really want it to work.
When you select shuffle what do you want to happen? What don't you want to happen?
Thanks!
Ben
This topic has been closed for further comments. You can use the search bar to find a similar topic, or create a new one by clicking Create Topic at the top of the page.
Page 4 / 9
class Shuffle{
private List<track> playlist;
private List<track> playedSongs = new List<track>();
public shuffle(List<track> playlist){
//initalises object and sets playlist
this.playlist = playlist;
}
public track GetRandomTrack(){
//gets the random track and adds it to the played songs
//this is random in terms of a different order and not played before, if all songs have been played
//it re-randomises it
int randomTrackNumber = GetRandomTrackNumber();
playedSongs.Add(randomTrackNumber);
return playlist[randomTrackNumber];
}
private int GetRandomTrackNumber(){
//gets the random track number
List<int> unplayedmap = getUnplayedTrackMap();
//if there isn't anything then everything has been played, reset the shuffle
if(unplayedmap.Count == 0)
{
ResetSuffle();
return GetRandomTrackNumber();
}
//gets the random unplayed track index to play and returns the actual track index
int trackIndexToPlay = getRandomInt(0, unplayedmap.Count);
return unplayedmap[trackIndexToPlay];
}
private void ResetSuffle()
{
//resets the shuffle by clearing what has been played
playlist.Clear();
}
private int getRandomInt(int min, int max)
{
//generate a random in between the two values
Random r = new Random();
decimal next = (decimal)r.NextDouble();
return (int)(min + (next * (min - max)));
}
private List<int> getUnplayedTrackMap()
{
//creates a list of unplayed tracks, it contains the index of the track in the playlist
List<int> unplayed = new List<int>();
for(int trackIndex = 0; trackIndex < playlist.Count; trackIndex++)
{
if (!playedSongs.Contains(playlist[trackIndex])){
unplayed.Add(trackIndex);
}
}
return unplayed;
}
}
talk about being proactive 🙂
You have to be kidding.
I spent $8,000 on Sonos, but i cant use the controller to play my playlists because i hear then same 1/50th of my music over and over when on random. Now i am forced to use iTunes to shuffle and input to Sonos as a TV in making the system a bunch of dumb speakers i can only control from my mac, not any handheld device. At least iTunes shuffles where Sonos does not appear to. If you were facing this issue, you would likely grow very tired of the 2 year wait for a solution. Just because you don't see a problem, does not mean others are being silly. If Sonos wanted to play real smart, they would release an SDK and let people help them evolve their software.
My wife, who is not technical gave a product review when i explained why she kept hearing the same few songs over and over: "well that's a bit crap then, isn't it"
yaay, any sort of timeline would be really appreciated!
wow good shuffle guys - just had a song repeat after 3 hours...... our playlist has 1600 songs!
Wait until you set a playlist for alarm with your 1600 songs and get woken by the same music every day...
Wait until you set a playlist for alarm with your 1600 songs and get woken by the same music every day...
haha yeah we scrapped the alarm ages ago.
about a year ago I decided to stop using the sonos shuffle and copy my spotify playlist into a randomizer, then into a new playlist and playing that in sonos. repeated the process when we finished all 1600
then last week i decided to go back to using the shuffle button..... i've changed my mind 🙂
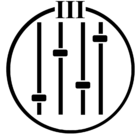
@jay.shellcrass
Try turning off the repeat function. With only shuffle on and not repeat, each song in your queue will play once. With repeat on, you'll potentially hear songs play multiple times.
repeat is not on so your comment is incorrect
Userlevel 1
That may be true John, but the songs will always play IN THE SAME ORDER when on shuffle, unless you start from a different song each time. Hence the whole thread.
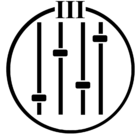
@Edward - this is true (as you say, hence the reason for this thread). The shuffle function operates by shuffling the songs in the queue, once shuffled the songs will always play in the same order. You can obtain a different order by toggling shuffle or by clearing your queue.
Userlevel 1
Your statement about "repeat function" is false. Shouldn't have to hit shuffle every time I play the que.
If I re-shuffle the queue then I will end up potentially with the same songs in my queue. It has been years. lets get something done.
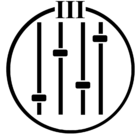
@Scott
The way shuffle works on Sonos is that hitting shuffle will randomly order all the tracks you have in the queue. Each track will play once, in that shuffled order, and then at the end playback will stop. With repeat on as well, that same ordering of shuffled tracks in the queue will loop.
What's confusing about all this is that the shuffled order isn't shown in the queue itself, the queue still shows you the normal ordering. Does this explanation make sense? You can verify it on your own system.
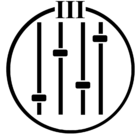
@Duncan Colby
You'll always end up with the same songs in the queue (until you remove them or add different ones). When you toggle shuffle (off and on again), you'll end up with a different shuffled order of those same tracks.
Try it - add an album to your queue and then hit shuffle. Start from the first track, and skip through a few songs. Then restart from the first track and skip a few times more. It's the same order because the shuffle function is creating a new ordering of the queue.
Now toggle shuffle and repeat the above. You'll end up with a different shuffled order of the same tracks.
Thanks John. That makes sense. But it doesn't explain why so many people on here are getting songs being played multiple times in one session. See Paul Keenan's post above (2 weeks ago). How does that happen?
Here is the issue. If you load the same playlist from an external service you get the same 'random' order each time. Why does loading that not shuffle? If I group my speakers play some songs and then ungroup one and then go to that one and play it will play the same songs I just heard on the others....just doesn't seem very random. How about showing the queue in the order they will play at least?
Userlevel 1
@ John M - No confusion - it just doesn't work the way you state.
John,
If you pause a shuffled playlist, what is supposed to happen when you un-pause?
Paul
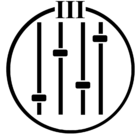
@Scott
If you're seeing something different, would you mind providing the steps for how you obtained those results?
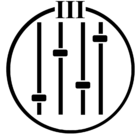
@Scott
If you're seeing something different, would you mind providing the steps for how you obtained those results?
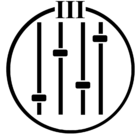
@Paul Richardson
When you pause, it will save that position in the currently playing track in its order in the queue. When you resume, it will start from that point in the track and that position in the queue. Pausing / resuming will not re-shuffle the queue.
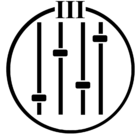
Hi, thanks for the comment. I don't think this is the case. Try adding a playlist from an external service with shuffle on and then skip forward a few times while noting the tracks that play. Then clear your queue and add the same playlist again, and repeat the play / skip. You should see a different order on the second time.
Userlevel 1
@ John M -
Sure - random is on... repeat is off. Start my day hitting the "mute" button on one of my devices... music plays thru the day - think to myself - I heard that yesterday. Hit "mute" again about 6:pm... wake up the next day - music plays thru the day - think to myself - I heard that earlier in the day... repeat.
My que has 7,338 tracks in it; besides maybe a studio and live track - there are no repeat songs in the que. That should be 15 days at 24/hr day listening; 30 days @ the 12 hours a day I listen to it.
I have just read John M's post and find it amazing (and worrying) that he does not at least touch on or even mention that a colleague (Ben) is working on the shuffle issue! I'm sorry, I just don't get it! Does John know Ben? Does left hand know what right hand is doing? Will we get a working shuffle? Who knows?
Page 4 / 9
Enter your username or e-mail address. We'll send you an e-mail with instructions to reset your password.